1. WAP to calculate the Factorial of a given number N in Flowchart, algorithm and C .
Algorithm:
Step 1: Start
Step 2: Declare Variable n, f, i
Step 3: Read number n from User
Step 4: Initialize Variable f=1 and i=1
Step 5: Repeat Until i<=number
Step 6: f=f*i
Step 7: i=i+1
Step 8: Print f
Step 9: Stop
Flow Chart:
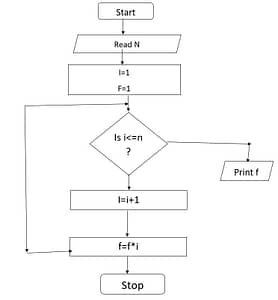
Code :
#include<stdio.h>
int main(){
int i,f=1,num;
printf(“Enter a number: “);
scanf(“%d”,&num);
for(i=1;i<=num;i++)
f=f*i;
printf(“Factorial of %d is: %d”,num,f);
return 0;
}
Output:
Enter a number: 10
Factorial of 10 is: 3628800
-
WAP to calculate the given number is Positive of Negative in Flowchart, algorithm and C .
Algorithm:
Step 1:Â Start
Step 2: Read a number n from user
Step3: If n is less than zero
Step4: Print The number is Negative number
Step5: Else if the number is greater than zero
Step6: Print The number is Positive number
Step7: Else Print the number is zero
Step8: End
Flow Chart:
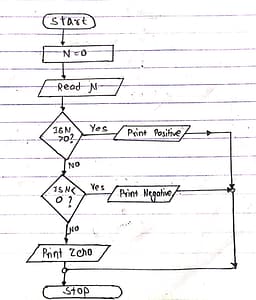
Code :
#include <stdio.h>
int main(){
 int num;
printf(“Input a number :”);
 scanf(“%d”, &num);
 if (num >= 0)
 printf(“%d is a positive number \n”, num);
 else
 printf(“%d is a negative number \n”, num);
return 0;
}
Output:
Input a number :4
4 is a positive number
3. WAP to find whether the input number is odd or Even in Flowchart, algorithm and C .
Algorithm:
Step 1: Start
Step 2: Read Number n
Step 3: If n mod 2 = 0 Then
Step4: Print n is an Even Number.
Step5: Â Else Print n is an Odd Number.
Step 6: Stop
Flowchart :
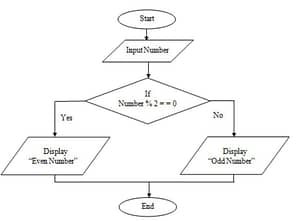
Code :
#include <stdio.h>
int main()
{
int num1, rem1;
printf(“Input an integer : “);
scanf(“%d”, &num1);
rem1 = num1 % 2;
if (rem1 == 0)
printf(“%d is an even integer\n”, num1);
else
printf(“%d is an odd integer\n”, num1);
return 0;
}
Output:
Input an integer : 4
4 is an even integer
4. WAP to take information of a student as input and display them suitably. Information includes name, roll no, age, phone number and email address in Flowchart, algorithm and C .
Algorithm:
Step 1: Start
Step 2: Declare variable name,roll no,age,phone number and email .
Step 3: Read name,rollno,age,phone number and email .
Step4: Print name,rollno,age,phone number and email .
Step5: Stop
Flow Chart :Â Â
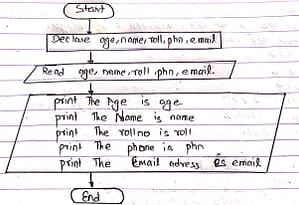
Code :
#include <stdio.h>
#include <conio.h>
int main(){
char nam[20];
int roll;
char adress[25];
int age;
int phon;
printf(“Enter The name “);
gets(nam);
printf(“Enter The Adress “);
gets(adress);
printf(“Enter the roll no”);
scanf(“%d”, &roll);
printf(“Enter the age”);
scanf(“%d”, &age);
printf(“Enter the Phone no”);
scanf(“%d”, &phon);
printf(“The name is %s\n”, nam);
printf(“The Adress %s\n”, adress);
printf(“The Roll no is %d\n”, roll);
printf(“The age is %d\n”, age);
printf(“The Phone number is %d\n”, phon);
return 0;
}
Output:
Enter The name kiran
Enter The Adress butwal
Enter the roll no20
Enter the age18
Enter the Phone no984855
The name is kiran
The Adress butwal
The Roll no is 20
The age is 18
The Phone number is 984855
4. WAP to swap two numbers input by uers in Flowchart, algorithm and C .
Algorithm :
Step1: Start
Step2: Declare a variable a,b and c as integer
Step3: Read two numbers a and b
Step4: c=a
Step5: a=b
Step6: b=c
Step7: Print a and b
Step9: End
Flowchart :
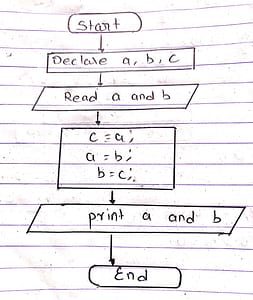
Code:
#include <stdio.h>
int main()
{
int a;
int b;
int c;
printf(“Input enter 1st Number”);
scanf(“%d”, &a);
printf(“Input Enter 2nd Number”);
scanf(“%d”, &b);
c = a;
a = b;
b = c;
printf(“The 1st number is %d\n”, a);
printf(“The 2ndst number is %d”, b);
return 0;
}
Output :
Input enter 1st Number5
Input Enter 2nd Number6
The 1st number is 6
The 2ndst number is 5
5. WAP to calculate compound amount when p n and r are given in Flowchart, algorithm and CÂ .
Algorithm:
Step1: Start
Step2: Declare a variable p,n and r and amount as integer
Step3: Read p,n and r
Step4: amount =p*[(1+r/n)^nt]
Step5: Print amount
Step6: End
Flowchart :
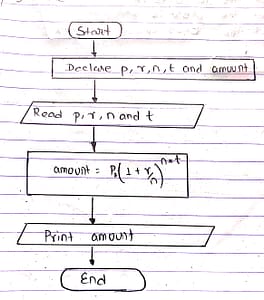
Code :
#include<stdio.h>
#include <math.h>
int main(){
float p,r,n,t,amount;
printf(“Enter principal”);
scanf(“%f”,&p);
printf(“Enter Rate”);
scanf(“%f”,&r);
printf(“Enter number of times interest”);
scanf(“%f”,&n);
printf(“Enter time”);
scanf(“%f”,&t);
amount = p* (pow((1 + r / n), t*n));
printf(“The Compount interest amount is %f”,amount);
return 0;
}
          Output:
Enter principal4500
Enter Rate2
Enter number of times interest1
Enter time2
The Compount interest amount is 40500.000000